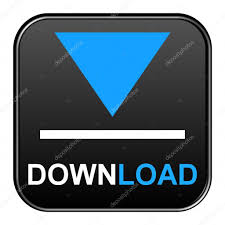
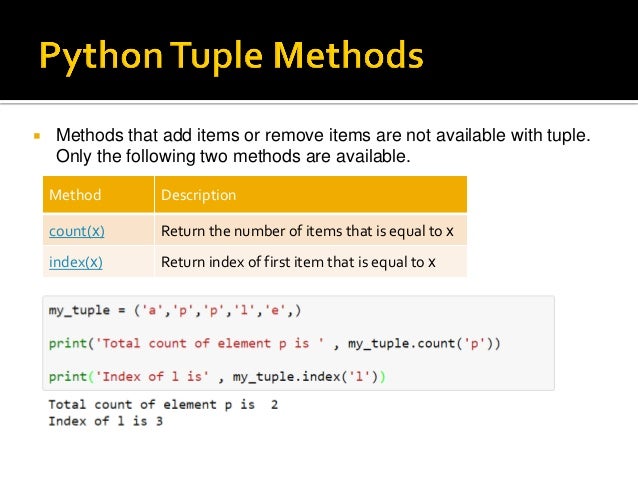
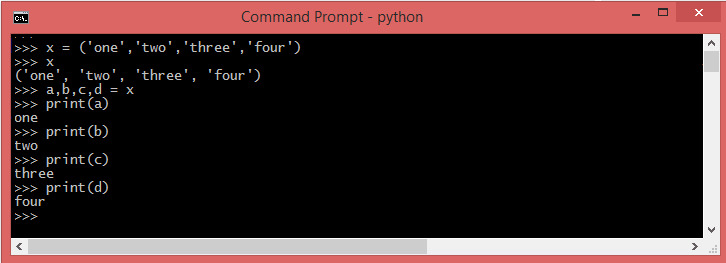
Output: Traceback (most recent call last): File "", line 1, in ValueError: not enough values to unpack (expected 5, got 4) Unpacking in Python is similar to unpack a box in real life. > director, scenarist, year, imdb_rating, stars = django_movie The right side is also a tuple of two integers 1 and 2.
Tuple unpacking python 3 code#
For example: x, y ( 1, 2) Code language: Python (python) The left side: x, y Code language: Python (python) is a tuple of two variables x and y. It says we tried to unpack a larger tuple into smaller numbers of variables. Unpacking a tuple means splitting the tuple’s elements into individual variables.

Output: Traceback (most recent call last): File "", line 1, in ValueError: too many values to unpack (expected 3) > director, year, imdb_rating = django_movie If the number of elements is either more or less, ValueError raises. Keep in mind that there must be the same number of variables equal to the length of the tuple. Of course, there are certain rules which have to be obeyed when using tuple unpacking. In fact, tuple unpacking has the same meaning with multiple assignment of a tuple in Python. > director, year, imdb_rating, stars = django_movieĪs you see, tuple unpacking is completely the opposite of tuple packing so that we assign each element of a tuple into multiple variables. Instead of doing so, there is a more Pythonic way to handle it: tuple unpacking. During unpacking, the number of variables must be equal to the number of objects in the. Let’s try to access elements of django_movie : > director = django_movie > year = django_movie > imdb_rating = django_movie > stars = django_movie Unpacking tuples assigns the objects in a tuple to multiple variables. We just assigned multiple variables, which are "Tarantino", 2012, 8.4 and "Waltz & DiCaprio", into a tuple, which is django_movie. A comparison operator in Python can work with tuples. Packing and Unpacking of Tuples In packing, we place value into a new tuple while in unpacking we extract those values back into variables. You have already used tuple packing because it is as simple as: > django_movie = ("Tarantino", 2012, 8.4, "Waltz & DiCaprio") Python has tuple assignment feature which enables you to assign more than one variable at a time. Tuple unpacking refers to assigning a tuple into multiple variables. If names correspond to single items of any sequence, then there must be enough names to cover all of the items. Each name on the left corresponds with either an entire sequence or a single item in a sequence on the right.

Then the variable first will be assigned ‘m’, the variable last will be assigned ‘a’, and the variable middle will just be an empty list since there are no other values to assign to it.Tuple packing refers to assigning multiple values into a tuple. Python has tuple assignment feature which enables you to assign more than one variable at a time. I find the Python 2 tuple unpacking pretty straightforward. If there are not enough values to unpack for the mandatory variables, we will get a ValueError.įor example, if we used the following assignment instead: first, *middle, last = ‘ma' The middle variable, due to using the * or unpacking operator, can have any number of values, including zero. Note: The first and last variables above are called mandatory variables, as they must be assigned concrete values. And the variable middle will contain all the letters between ‘M’ and ‘l’ in the form of a list. The last letter, ‘l’, is assigned to the variable last. Lists are mutable, and their elements are usually homogeneous and are accessed by iterating over the list. As such, the first letter of ‘Michael’ is assigned to the variable first, which would be ‘M’ in this case. Tuples are immutable, and usually contain a heterogeneous sequence of elements that are accessed via unpacking (see later in this section) or indexing (or even by attribute in the case of namedtuples ). The values on the right side of the assignment operator will be assigned to the variables on the left depending on their relative position in the iterable object. We can do so as follows: first, *middle, last = nameĪnd that’s it! Since name is a string, and strings are iterable objects, we can unpack them. Let’s say that we have a string assigned to the variable name: name = ‘Michael’Īnd we want to break this name up into 3 parts, with the first letter being assigned to a variable, the last letter being assigned to another variable, and everything in the middle assigned to a third variable.
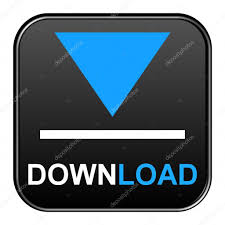